Adding a Map to your App
For many geospatial applications you will want to add a map to your Fused App, especially if your UDF returns a Map Tile
.
This section shows a few examples of how you can do that. While we do recommend you use pydeck
(the Python implementation of deck.gl) for its versatility, you can use other options like folium
Note that you need to install dependencies with micropip
inside your Fused app. More on this here.
Pydeck
Create a pydeck TileLayer
that plots a simple GeoDataFrame.
You can find this app right here and test it for yourself!
Here's what this would look like:
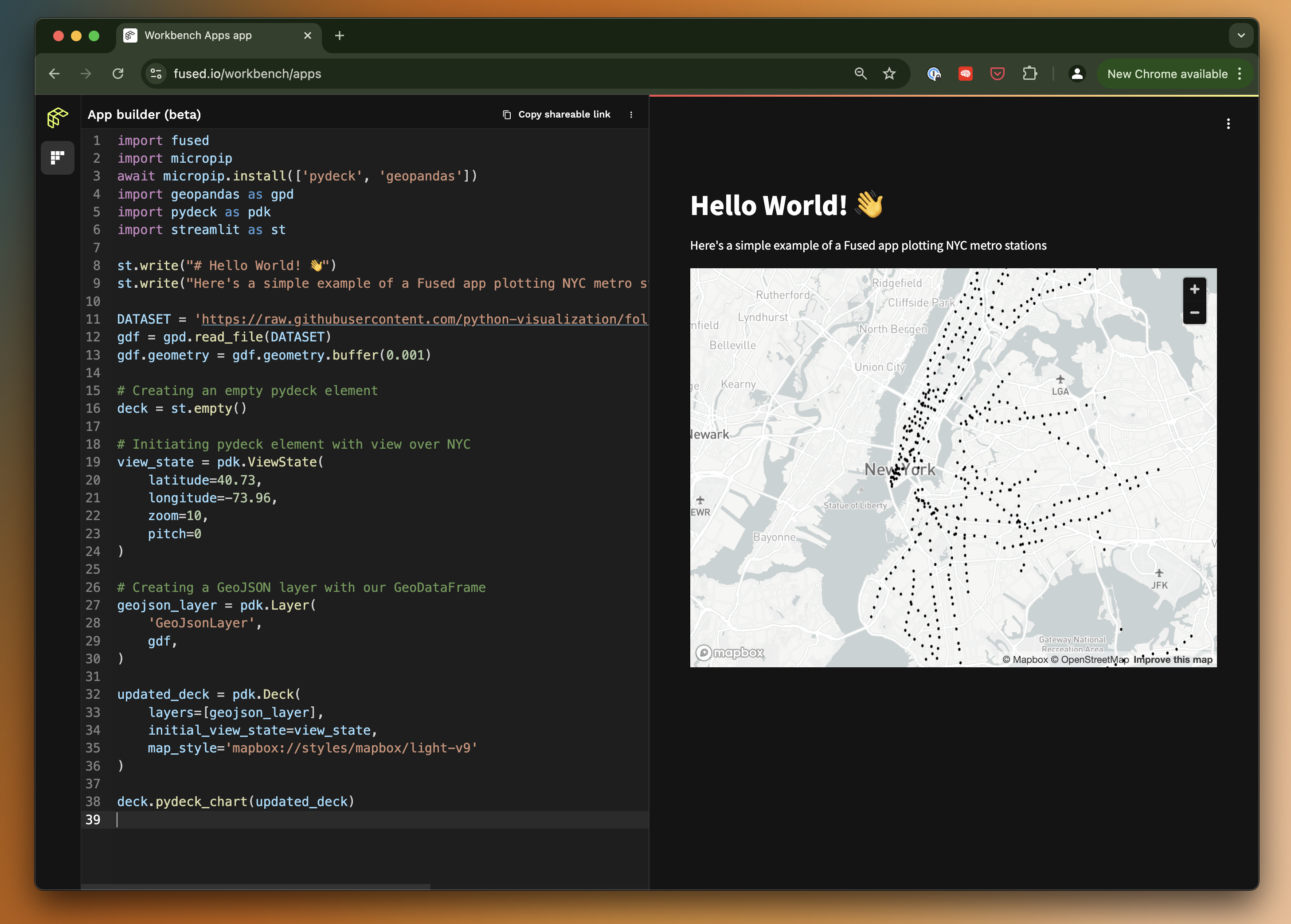
\
# installing pydeck & geopandas inside Fused app
import micropip
await micropip.install(['pydeck', 'geopandas'])
import fused
import geopandas as gpd
import pydeck as pdk
import streamlit as st
st.write("# Hello World! 👋")
st.write("Here's a simple example of a Fused app plotting NYC metro stations")
DATASET = 'https://raw.githubusercontent.com/python-visualization/folium-example-data/main/subway_stations.geojson'
gdf = gpd.read_file(DATASET)
# We buffer the points to make them more visible on our map
gdf.geometry = gdf.geometry.buffer(0.001)
# Creating an empty pydeck element
deck = st.empty()
# Initiating pydeck element with view over NYC
view_state = pdk.ViewState(
latitude=40.73,
longitude=-73.96,
zoom=10,
pitch=0
)
# Creating a GeoJSON layer with our GeoDataFrame
geojson_layer = pdk.Layer(
'GeoJsonLayer',
gdf,
)
updated_deck = pdk.Deck(
layers=[geojson_layer],
initial_view_state=view_state,
map_style='mapbox://styles/mapbox/light-v9'
)
deck.pydeck_chart(updated_deck)
Read more about how to use PyDeck on their official documentation
This example shows how to plot a GeoDataFrame
directly, but you could swap this out for a UDF that returns a GeoDataFrame
too:
# DATASET = 'https://raw.githubusercontent.com/python-visualization/folium-example-data/main/subway_stations.geojson'
# gdf = gpd.read_file(DATASET)
gdf = fused.run("YOUR_UDF_RETURNING_A_GDF")
Read more about fused.run
in the dedicated section
Folium
Create a streamlit-folium TileLayer
that calls a UDF HTTP endpoint.
import folium
from streamlit_folium import st_folium
m = folium.Map(location=[22.5, -115], zoom_start=4)
url_raster = 'https://www.fused.io/server/v1/realtime-shared/fsh_3QYQiMYzgyV18rUBdrOEpO/run/tiles/{z}/{x}/{y}?dtype_out_raster=png'
folium.raster_layers.TileLayer(tiles=url_raster, attr='fu', interactive=True,).add_to(m)
st_folium(m)