Map Tile/File
When UDFs are called, they run and return the output of the execution. They can be called in two ways that influence how Fused handles them: File
and Tile
.
Single File
In File
mode, the UDF runs within a single HTTP response. This is suitable for tasks that can be completed in a single request, such as processing data that fits in memory.
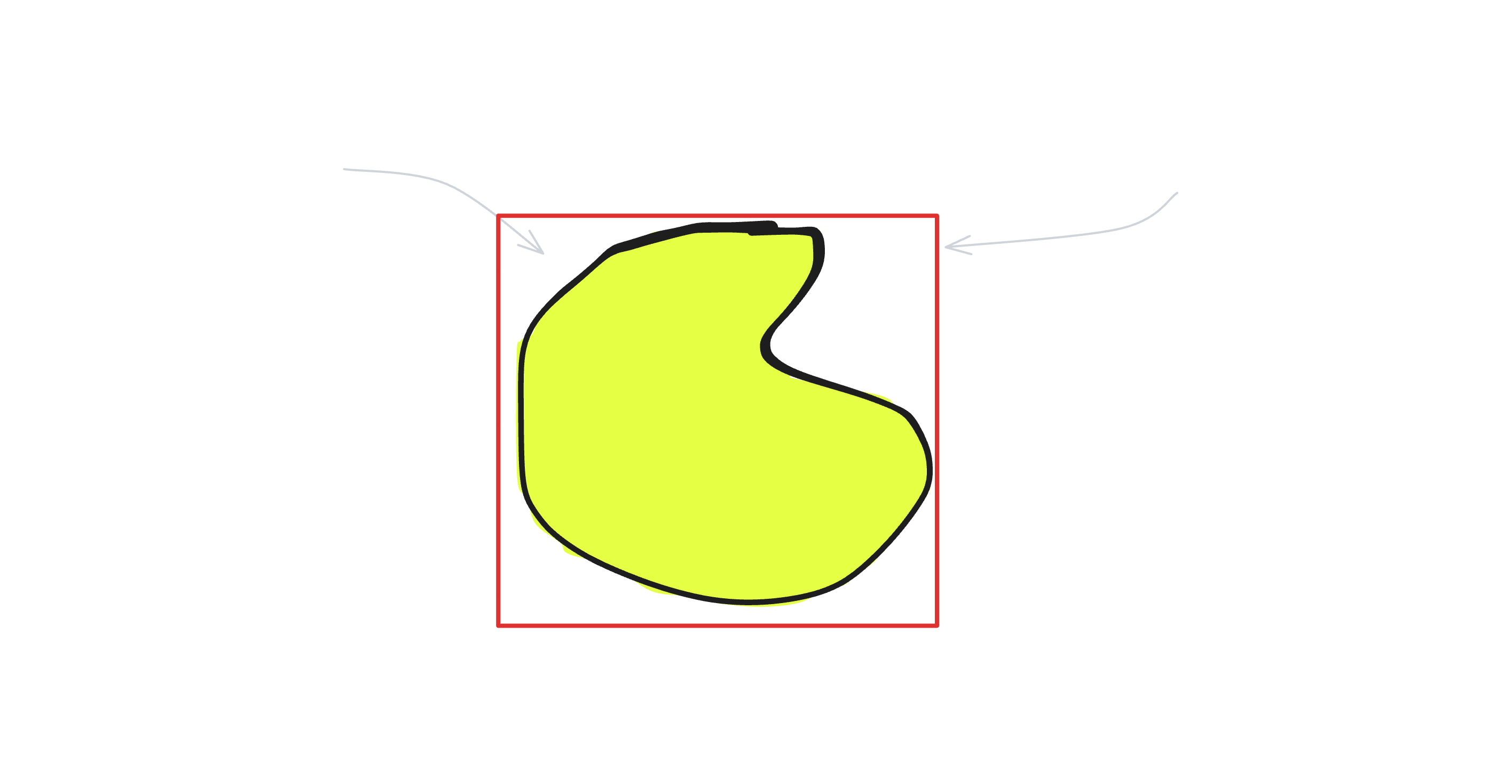
Map Tiles
Tile
mode is designed to process geospatial datasets in grid-based tiles that align with web map tiling schemas. Each Tile request may correspond to a spatial slice of a larger dataset, making it ideal to work with large datasets that can be spatially filtered.
When a UDF endpoint is called as Tile
, Fused passes bbox
as the first argument, which the UDF can use to spatially filter the dataset. The bbox
object specifies a tile by its bounds or XYZ index.
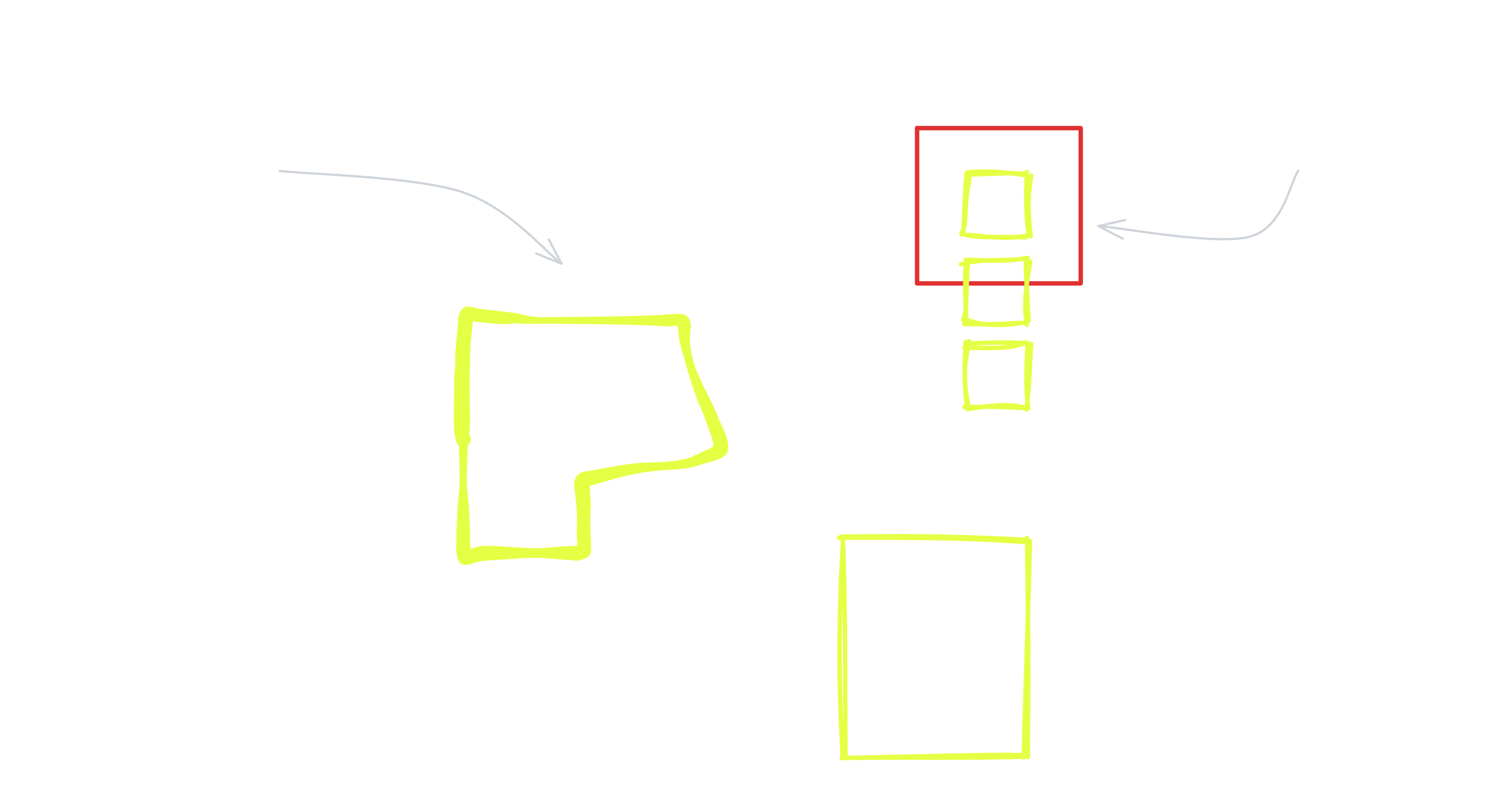
This is in contrast with a File
call, where the UDF runs within a single HTTP response. In File
mode, the UDF doesn't receive a bbox
object to spatially filter data into tiles.
Responses with spatial data can render on a map. GeoDataFrames
already contain spatial geometry information. If a raster does not contain spatial information, the bounds must be specified alongside the output object, separated by a comma, to determine its location on a map.
return arr, [xmin, ymin, xmax, ymax]
The bbox
object
A UDF may use the bbox
parameter to spatially filter datasets and load into memory only the data that corresponds to the bbox
spatial bounds. This reduces latency and data transfer costs. Cloud-optimized formats are particularly suited for these operations - they include Cloud Optimized GeoTiff, Geoparquet, and GeoArrow.
bbox
object types
The bbox
object defines the spatial bounds of the Tile, which can be represented as a geometry object or XYZ index. For convenience, a user writing a UDF can select from 3 bbox
object structures using a parameter type.
fused.types.TileGDF
This is a geopandas.geodataframe.GeoDataFrame with x
, y
, z
, and geometry
columns. UDFs use this as default if no type is specified.
@fused.udf
def udf(bbox: fused.types.TileGDF=None):
print(bbox)
return bbox
>>> x y z geometry
>>> 0 327 790 11 POLYGON ((-122.0 37.0, -122.0 37.1, -122.1 37.1, -122.1 37.0, -122.0 37.0))
fused.types.Bbox
This is a shapely.geometry.polygon.Polygon corresponding to the Tile's bounds.
@fused.udf
def udf(bbox: fused.types.Bbox=None):
print(bbox)
return bbox
>>> POLYGON ((-122.0 37.0, -122.0 37.1, -122.1 37.1, -122.1 37.0, -122.0 37.0))
fused.types.TileXYZ
This is a mercantile.Tile object with values for the x
, y
, and z
Tile indices.
@fused.udf
def udf(bbox: fused.types.TileXYZ=None):
print(bbox)
return bbox
>>> Tile(x=328, y=790, z=11)
Call HTTP endpoints
A UDF called via an HTTP endpoint is invoked as File
or Tile
, depending on the URL structure.
File endpoint
This endpoint structure runs a UDF as a File
. See implementation examples with Felt and Google Sheets for vector.
https://www.fused.io/server/.../run/file?dtype_out_vector=csv
Tile endpoint
This endpoint structure runs a UDF as a Tile
. The {z}/{x}/{y}
templated path parameters correspond to the Tile's XYZ index, which Tiled web map clients dynamically populate. See implementation examples for Raster Tiles with Felt and DeckGL, and for Vector Tiles with DeckGL and Mapbox.
https://www.fused.io/server/.../run/tiles/{z}/{x}/{y}?&dtype_out_vector=csv
Call fused.run
A UDF called with fused.run
runs as Tile
when the Tile
geometry is specified with reserved parameters in the following ways. Otherwise, it runs as File
.
Specify a Tile's bounds with a gpd.GeoDataFrame
or shapely.Geometry
as the bbox
parameter.
fused.run(my_udf, bbox=bbox)
Specify a Tile by its index in the x
, y
, and z
parameters.
fused.run(my_udf, x=1, y=2, z=3)